That sounds really nice already 
But does that use the physics library of the OS 3.2 ?
No I made all by myself and so it can work on any "Lua supporting OS" !

Nice !
Levak and I were actually creating one using physics too x)
(started like 3 days ago)
Edit : 3.2's Physics Engine.
Really ? ^^
Yes the 3.2's Physics Engine could be usefull for such a project !

Please could you helpme understand the physics o theballs?
Collisions with walls aren't very difficult, but between balls it's kinda complicated..
Each ball is characterized by its coordinates and velocity. (no use to consider any acceleration) So I have a table like this which contains these informations for each ball :
balls={{x1,y1,vx1,vy1},{x2,y2,vx2,vy2},...,{xn,yn,vxn,vyn}}
First of all, you have to consider all couple of balls which could collide and test if they collide. The number of couple is thereby "2 among n". (nCr(n,2), binomial coefficiant)
For example the list of collision for 4 balls is :
{{1,2},{1,3},{1,4},{2,3},{2,4},{3,4}} (6 collision possibilities = nCr(4,2))
Now, lets consider two balls wich could collide :
ball1 with x1,y1,vx1,vy1
ball2 with x2,y2,vx2,vy2
Their radius is r.
They collide if (x2-x1)^2+(y2-y1)^2 < (2*r)^2.
If they do, we have to calculate the new velocity of each ball.
By velocity subtraction, we can bring us to a more simple problem with :
- the ball 2 moving at the velocity {vx2-vx1,vy2-vy1}
- the ball 1 wich is immobile.
Now, after thinking, that's how I modeled the behavior of a moving ball hitting an other wich is motionless :
- the direction of the new velocity of the ball 1 is collinear with the vector defined by the center of gravity of each ball. (we will call this vector g2g1)
That's it :
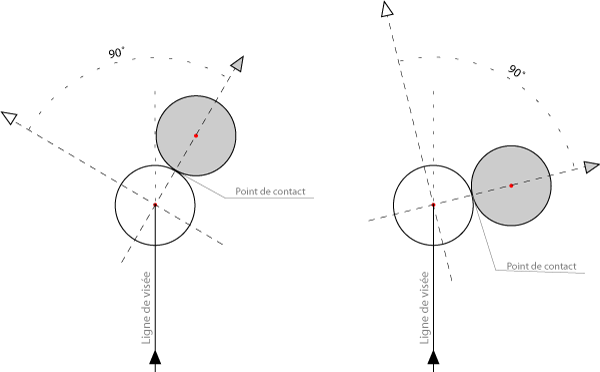
from wikipédia
About the norm of the new velocity of the ball 1 (v1) : if v2 is the velocity of the moving ball (ball 2) and a is the angle between the direction of the vector g2g1 and the vector v :
v1 = v2*cos(a)
Thus, for example, if you hit the ball in the center, g2g1 and v are colinear and cos(a)=1 and the entire velocity of ball 2 is transmitted to the ball 1.
If they touch softly when g2g1 and v are almost perpendicular, ball 1 won't move much.
So we have defined the new velocity of ball 1 and to have the velocity of ball 2 (the moving ball) you just have to subtract the velocity transmitted to the ball 1.
v2=v2-v2*cos(a)
(I haven't considered loses of power in ball-ball collisions since the coefficient of restitution between two balls is pretty high, and I don't want to do useless calculations..) However I have considered friction with table and a restitution of 75% in a ball-wall collision.

So if we want these results in the case where ball 1 and ball 2 have their own velocity, we can have this result :
v1=v1+norm_v*cos(a)
v2=v2-norm_v*cos(a)
where v1 and v2 are vectors and norm_v = |v2-v1|
and as we want this on x/y, we have :
vx1=vx1+norm_v*cos(a)*cos(b)
vy1=vy1+norm_v*cos(a)*sin(b)
where b is the angle between Ox axis and the vector v1, which is colinear, as we said before, with the vector g2g1.
vx2=vx2-norm_v*cos(a)*cos(b)
vy2=vy2-norm_v*cos(a)*sin(b)
But how to calculate a and b angles ? (actually, cos(a), cos(b) and sin(b))
With the scalar product and so on we can have very good simplifications ! (no cos, no sin, no norm_v

)
And here is how looks the program for this part :
gx=boul[combi[i][2]][1]-boul[combi[i][1]][1]
gy=boul[combi[i][2]][2]-boul[combi[i][1]][2]
norm_g=gx^2 + gy^2
if math.floor(math.sqrt(norm_g)) < 2*r then
vx=boul[combi[i][2]][3]-boul[combi[i][1]][3]
vy=boul[combi[i][2]][4]-boul[combi[i][1]][4]
scal=gx*vx+gy*vy
boul[combi[i][1]][3]=boul[combi[i][1]][3]+math.floor(scal*gx/norm_g) -- vx + norm_v*cos((g,v))*cos((Ox,g))
boul[combi[i][1]][4]=boul[combi[i][1]][4]+math.floor(scal*gy/norm_g) -- vy + norm_v*cos((g,v))*sin((Ox,g))
boul[combi[i][2]][3]=boul[combi[i][2]][3]-math.floor(scal*gx/norm_g)
boul[combi[i][2]][4]=boul[combi[i][2]][4]-math.floor(scal*gy/norm_g)
end
It's a bit confuse but I hope you got some things.. ^^
So this is how I modelized collisions ! I don't know if it's right but it seems to be not bad at all !
