This section allows you to view all posts made by this member. Note that you can only see posts made in areas you currently have access to.
Messages - Quigibo
Pages: 1 ... 126 127 [128] 129 130 ... 135
1906
« on: February 28, 2010, 01:44:25 am »
That's already been done. It will be in the next release coming very soon. You have to manually store data though since I haven't added automated data structure support yet. I'll explain what I mean by that when I release it, but basically you have to keep track of the size of the array, dimensions of the array, and maximum size of the array yourself.
1907
« on: February 28, 2010, 01:35:32 am »
That would be pretty cool! But Axe syntax is really designed around the limitations of the tokens available to Basic programmers as well as z80 itself. If the Nspire is not token based and uses ARM assembly, it would be easier just to create a C language or something well defined since its more universal and there would be more support for it. You would have to start from scratch either way since this isn't something that would be "portable" since it is so heavily dependent on z80 and token parsing.
I might get an Nspire myself after its finally cracked, but until then, I'm just going to stick with z80.
1908
« on: February 27, 2010, 09:23:58 pm »
Safe Copy requires the undocumented instruction: in f,(c)
Will that be compatible with the Nspire? If anyone has one, could you please try adding this to any Axe Parser code and see if it crashes:
Asm(0E10ED70)
1909
« on: February 27, 2010, 03:05:02 am »
Unfortunately no, I haven't been able to spot a pattern in the errors yet, but I haven't done a rigorous debugging either. I'll do that tomorrow probably.
1910
« on: February 26, 2010, 04:01:17 pm »
Sure. Lets say you do this:
<asm code 1> ld hl,(var_A) <asm code 2> ld (var_A),hl <asm code 3>
You can do: Asm(asm code 1):A Asm(asm code 2):->A Asm(asm code 3)
More generally, the variables are stored in saveSScreen in alphabetical order and then the random seed immediately follows.
1911
« on: February 26, 2010, 03:52:51 pm »
I'm going to post most of the assembly routines I use in Axe Parser here to see if any of you asm programmers can help me with their optimizations. - I am always trying to optimize for size, not speed, unless it is significantly faster and close to the same size.
- I would greatly prefer that it does not use any extra RAM to store temporary variables, but that's not that big of a deal.
- No self modifying code or undocumented commands because I need to make this compatible for Apps and with the Nspire TI-84 emulator.
The most important thing right now is the clipped sprite routine since its really big. Here's what I got so far: p_DrawOr8x8: push hl pop ix ;Input hl = Sprite ld b,7 ;Input c = Sprite X Position ld d,0 ;Input e = Sprite Y Position ld h,d ld a,c add a,b jr c,__ClipLeft sub 96+7 ret nc cpl cp b jr nc,__NoClipH __ClipRight: inc d jr __ClipHDone __ClipLeft: add a,89 ld c,a __ClipHDone: inc d ;d,c,e are updated __NoClipH: ld a,e add a,b jr c,__ClipTop sub 64+7 ret nc cpl cp b jr nc,__NoClipV jr __ClipBottom __ClipTop: inc ix inc e jr nz,__ClipTop __ClipBottom: ld b,a __NoClipV: ;b,ix,e are updated. dec d jr z,__NoFix inc e __NoFix: push de ld l,e ld d,h add hl,hl add hl,de add hl,hl add hl,hl ld e,c ld a,e srl e srl e srl e add hl,de ld de,plotSScreen-11 add hl,de pop de inc b and %00000111 jr z,__DrawOr8x8Aligned ld c,a __DrawOr8x8Loop: push bc ld b,c ld c,(ix+0) xor a __DrawOr8x8Shift: srl c rra djnz __DrawOr8x8Shift dec d jr z,__SkipRight or (hl) ld (hl),a __SkipRight: dec hl inc d jr z,__SkipLeft ld a,c or (hl) ld (hl),a __SkipLeft: ld c,13 add hl,bc inc ix pop bc djnz __DrawOr8x8Loop ret __DrawOr8x8Aligned: dec hl ld de,12 __DrawOr8x8AlignedLoop: ld a,(ix+0) or (hl) ld (hl),a inc ix add hl,de djnz __DrawOr8x8AlignedLoop ret __DrawOr8x8End:
If you spot anything that can be optimized, bold it so I can see what you changed, thanks!
1912
« on: February 26, 2010, 03:27:59 pm »
Ans is always implied as the last computation every new line so its not really needed. Storing it to a temporary variable is what "Ans" would do anyway, so just pick a variable and pretend that its "Ans".
I'm a little bit confused why you store to B and never use it and then reference T but don't declare it. I'll assume thats a typo and are meant to both be the same variable.
Here are some optimizations:
Lbl S ->A^64/16->B A^16->A If B^2 ;Says "If B is odd" 16-A->A End A-(A*A*A/600)->A If B/2^2 ;Checks 2nd bit -A->A End A Return This should now work at ANY value of A, but I guess it is still just one term of the Maclaurin so estimates will be off...
EDIT: oops, fixed.
1913
« on: February 26, 2010, 03:06:36 pm »
You'll have direct access to the screen ram in the next version so it will probably be a lot easier to do many of those effects.
By the way, I don't think I mentioned how to do masking. If Pic1 is the inverse of the sprite and the mask of the sprite is immediately after it, then to mask the sprite, you do:
:Pt-On(X,Y,Pic1+8) :Pt-Change(X,Y,Pic1)
What I mean by double buffering is that you can draw a background, like a tile map for instance, and then copy it to the extra back-buffer using "StorePic". When you want to draw over the buffer, then instead of using "ClrDraw", you use "RecallPic" to copy the extra buffer to the main buffer and then you only need to draw the foreground over that instead of having to render the entire foreground and background each time and then clearing it each time a sprite moves. I don't think it would be fast enough for flickerless gray scale like Pyoro, but flickerless gray scale support is planned in the future. By flickerless I literally mean solid gray, no faint crosshatches. Quality depends on how long it takes between renders, but its a reasonable amount of time you'll have to do basic rendering and computation. I'm sure you can get some quality of grayscale without the native support in the mean time though.
1914
« on: February 25, 2010, 05:20:09 pm »
Wow! Those are all really nice! That's way more than I thought would be possible to do already without ram access!
A little update on my progress... I've already added most of the features I was planning on, but it turns out that the built in data structure system is a lot more complicated than I though. You'll just have to make your own, which isn't really that hard, since I'm going to be adding other features in the mean time instead. Double buffer support is one of those things I might include. Basically its just a command to swap the buffer with the backup buffer so you can draw to each one separately.
1915
« on: February 24, 2010, 06:25:27 pm »
Yeah, I could, but it would be so inefficient. That would be like a BASIC programmer trying to make a compiler for assembly language programs, which I have seen done before, but its very slow. However, I could just cheat and the whole program would just be asm([hex code of entire program]) That would work  Safety features will come in due time. I'm kind of in that awkward phase where the next version about to come out has enough features to compile nearly any type of game you can think of, yet I still don't have a GUI (even the list of programs is awful because of the scrolling and such), no safety features like archiving the ram before testing the program, or a compiler options menu for things like 15MHz mode options. And there are still hundreds of commands I still have to add! I promise. After the next release, Axe Parser will become 0.1.x and make more of those changes instead of new commands and such.
1916
« on: February 24, 2010, 06:11:52 pm »
That's actually 1 byte less optimized. Try it.
That's because the parenthesis require a push and pop (2 bytes), and the "greater than" and "less than or equal to" operators take 2 more bytes than the other comparisons. So you saved 3 bytes but then added 4.
You could have done this:
:B>=(+D->B-1)+A+C->A But then its the same size as your first attempt. Not sure if the speed is faster or slower though.
1917
« on: February 24, 2010, 01:53:37 pm »
Nice! Although you don't need all those parenthesis since order of operations is still just left to right: :B+D->B<D+A+C->A :A-C-(B-D->B>=-D)->A Won't make a difference in the compiled code, but saves some memory in the source.
1918
« on: February 23, 2010, 10:17:38 pm »
You guys all complain about "RAM clears" and "corrupted memory". Have you ever tried to program in assembly? There are so many leaks and corruptions that happen, not to mention all the frustration of trying to find logical errors in the code, that almost all ASM programmers run their works in progress on emulators just in case and to speed up the process. I can guarantee that if you tried to make the same program in assembly (unless you're highly experienced with it), you would have had at least an order of magnitude more problems with the code.
Remember, me giving you the freedom to run lower level assembly code and commands is a trade-off with the safety of running it.
Now, if you get an unexpected error either at compile time or at run time, I really need to know about that so I can fix it. I currently am aware of 2 mysterious bugs. One is that the compiler sometimes gives a random error if the program was just modified but parsing a second time always works. I have no idea what is causing that. Another error mysteriously vanished when I added some code unrelated to the error, so it will probably come back to haunt me sometime. I think it had something to do with automatic parenthesis in math operations.
1919
« on: February 23, 2010, 07:05:52 pm »
Right, I haven't clipped the sprites yet, so that means it actually draws the sprite into the memory immediately after the buffer which contains a lot of important information the calc needs... so it will corrupt that data and do a RAM clear most likely. Might be fixed in the next version.
1920
« on: February 23, 2010, 04:56:13 pm »
I made something that was inspired by the Star Demo:
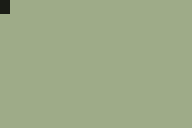
I used inline ASM to access my array of bullets, in case anyone is wondering how on earth I did that.
Hey, that looks very similar to the next example program I'm writing to test out the new features I'm adding. Though mine has enemies, collision detection, animated explosions, and even a story line so far
Pages: 1 ... 126 127 [128] 129 130 ... 135
|